The Death of Human-Written Code Tutorials in the ChatGPT Era … Or Not?
The Death of Human-Written Code Tutorials in the ChatGPT Era … Or Not?
An argument in favor of human-written coding tutorials in the new age of LLMs
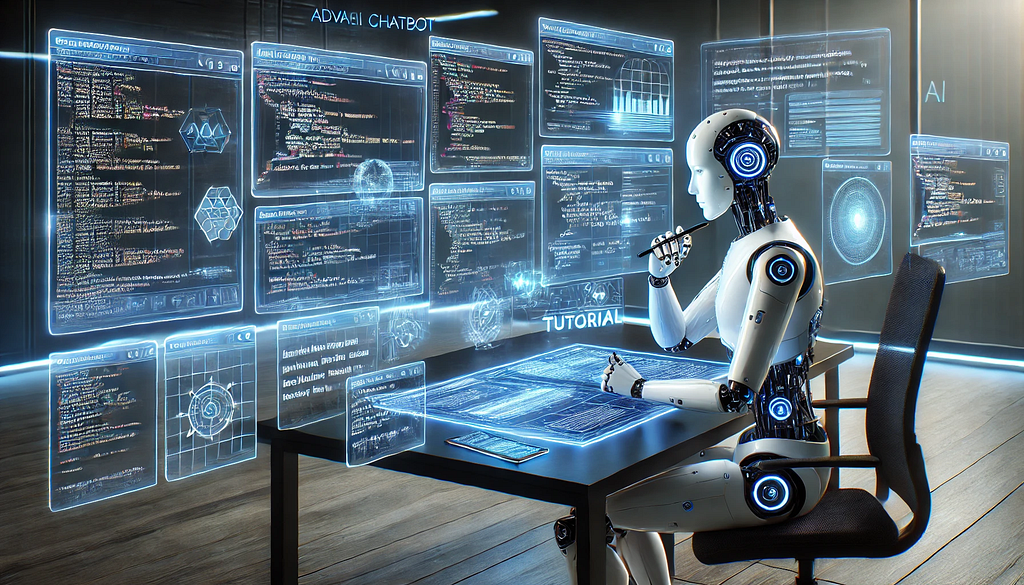
In a world where ChatGPT reigns, one might expect human-written code tutorials to fall flat.
After all, who’s going to read a human-written tutorial on a detailed software process, when they can simply ask ChatGPT for precisely the output they want? Toss in an advanced LLM subscription and a few advanced prompt engineering techniques, and anyone can generate detailed explanations about any topic. Why waste the time and effort to research, brainstorm, and write such an article?
Let’s begin with a fundamental question, the answer to which will underpin the entirety of my argument: what exactly is ChatGPT?
Well, ChatGPT, or any large language model (LLM) for that matter, operates via a machine learning algorithm that predicts what words should follow an input based on training data. What separates an LLM from earlier iterations of language models is the sheer size of its training data. ChatGPT’s training, for instance, included the Common Crawl data set — a giant data set that includes billions of web pages.
This is crucial to remember. No matter how incredible and seamless these generated responses appear, they are fundamentally just a probabilistic prediction grounded in prior data. They are the most likely output based on what the model has seen before. They are not novel.
Human creativity, by contrast, often results from new insights — commonly referred to as “light-bulb moments.” Yes, having prior knowledge helps — but at its core, the beauty of these new ideas is that they are precisely that: new. When an scientist or writer or artist has a rare moment such as this, they’ve hit the jackpot.
Let’s tie this back into the topic at hand: human-written coding tutorials. When do people write articles or publish books on a programming topic? Usually, for one of the following two reasons:
- To offer a new perspective on an existing idea. Many tutorials teach the same general topic, but with variations in style, approach, or methodology.
- To convey a new idea or topic altogether.
An LLM-generated response can do neither of these.
Let’s consider an example. In early 2023, I wrote “4 Essential Techniques You Must Learn as a Python Beginner” for Towards Data Science, an article that was generally well read and received. Below, I’ve inserted two excerpts:
- The section on lambda functions from the article I actually wrote.
- The section on lambda functions as written by ChatGPT-4o after I provided the necessary details and requested the associated content.
Example 1: A Lambda Tutorial by a Human
Let’s say you are working with some data in a Jupyter notebook, just doing some quick exploration and analysis. You’re still in the early stages of data cleaning and processing, far from any production-ready models or visualizations or applications. But you do have a deadline to meet, so you’re exploring quickly and efficiently, making use of your stellar Python skills.
In the midst of your adventures, you come across a column in your data that requires transformation. You just need to square the numbers in the column. It’s nothing crazy, but it’s unfortunately also one of those weird necessities that is simple enough to be quick, but complex enough to not have its own built-in function.
So, you decide to use pandas’s apply function to transform the data column using your own, custom function. To do this, you need to write a function that squares numbers, and you do so in the only way you know how:
def square(num):
return num * num
This gets the job done, but it’s a little annoying and messy, especially for a Jupyter notebook. It doesn’t meld well with the one-line structure of most pandas operations, and accordingly won’t look very nice when your colleagues review your notebook.
But do not despair, my friend, for the lambda function is here to save you. Lambda functions — or, more generally, anonymous functions — provide an alternative way to define functions in Python. And the best part is, you can write them in a single line! This is most easily seen via example:
square = lambda num: num * num
The above code is identical to our earlier definition of this function. Here are a few things you should know:
- The lambda keyword is analogous to the def keyword, letting Python know we want to define a function.
- The parameters for lambda functions are to the left of the colon, and the return statement is to the right of the colon (and doesn’t actually use the return keyword).
- We don’t have to give the function a name, but if we wish to do so, we can do it using variable assignment.
That last point is key. Using lambda functions lets us define a function and call it or pass it in as an argument to another function without ever having to give it a name. Let’s illustrate this by returning to our previous example and making it concrete.
Let’s imagine we have the DataFrame my_df below, containing three people’s salaries:
Name Salary
0 John 45000
1 Mary 60000
2 Julie 100000
In this ridiculously idealistic world, employers have just announced that everyone’s salaries will be squared. We can update our DataFrame in a single line by using a lambda function:
>>> my_df['Salary'] = my_df['Salary'].apply(lambda num: num * num)
>>> my_df
Name Salary
0 John 2025000000
1 Mary 3600000000
2 Julie 10000000000
And voila — endless riches! Perhaps a bit dramatic, but hopefully you will now remember what lambda functions are and how to use them. If you would like a more detailed discussion on their nuances and subtleties, I recommend checking out my two-part article series that discusses lambda functions in great detail.
Example 2: A Lambda Tutorial by a Large Language Model
Prompt: I am writing an article called “4 Essential Techniques You Must Learn as a Python Beginner” for the publication Towards Data Science on Medium. The 4 topics I will cover are 1) lambda functions, 2) list comprehensions, 3) continue and break statements, and 4) user input. Can you generate the section on lambdas for me? It should be about 300 words, consist of an example, and have a personable tone without being too casual.
Lambda Functions: Unlocking Python’s Anonymous Heroes
Lambda functions, also known as anonymous functions, are one of Python’s most flexible tools. While they might sound intimidating at first, their purpose is simple: to create small, single-use functions without the need for the usual def keyword or a function name. Think of them as quick, disposable tools in your Python toolbox.
Let’s break it down. A lambda function is defined with the keyword lambda, followed by the parameters, a colon, and the expression to evaluate. Here's the syntax:
lambda parameters: expression
Why use them? Lambda functions shine in situations where you need a simple function for a short period, such as when working with built-in functions like map(), filter(), or sorted().
Here’s an example to illustrate:
# Sort a list of tuples by the second element using lambda
data = [(1, 'apple'), (3, 'banana'), (2, 'cherry')]
sorted_data = sorted(data, key=lambda x: x[1])
print(sorted_data)
Output:
[(1, 'apple'), (3, 'banana'), (2, 'cherry')]
In this snippet, the lambda function lambda x: x[1] extracts the second element of each tuple, allowing sorted() to arrange the data alphabetically.
While lambda functions are incredibly handy, they come with a caveat: readability. Overusing them or cramming complex logic into a lambda can make your code harder to understand. When your logic gets more complex, it’s better to stick to regular functions with descriptive names.
In essence, lambda functions are a quick way to write short, throwaway code. As a beginner, mastering them will not only make your code cleaner but also help you better understand how Python embraces simplicity and power.
Let’s break down some important differences between these two excerpts. In each bullet that follows, I describe the difference, and then explain why it is important.
- The human version opens with a motivating problem, whereas the AI version gets straight into definitions. People love examples. They make difficult concepts easier to understand and make writing more engaging. It’s worth pointing out that you could ask an AI to start with an example, but that misses the fundamental point: unlike high-quality human writers, AI by default does not know how to effectively curate and fine tune writing to achieve the best results with varied audiences. Not starting this particular excerpt with a motivating opening is just one example of this broader reality.
- The AI-generated version fails to address the fact that lambda functions can be assigned to a variable if one wishes to reuse them, and also confines them to a single use with the sentence, “In essence, lambda functions are a quick way to write short, throwaway code.” Both of these flaws — the former an omission of important information, and the latter a needlessly absolutist statement — have the potential to mislead the novice programmers who are often the target audience for code tutorials. A reader should be aware that there is a way to prevent lambda functions from immediately disappearing, and they should also understand that while lambdas can sometimes be used as a throw-away functions, they can also be a useful tool in certain programming environments (such as within a functional paradigm). Or, even if the details of this are unnecessary to describe for beginners, a tutorial definitely should not explicitly state that lambdas are essentially always throw-away functions.
- Something about the writing in the AI version feels off, but it is difficult to pinpoint what exactly that is at first glance. If you were to read and break down the two excerpts carefully — as one might do in a writing/rhetoric course — you would realize that the human version flows better and is easier to follow. The reason for this is that the human version revolves around a single thread — working with a data set and using lambda functions to accomplish a specific goal more effectively — which stretches through the entire excerpt. Everything else, including the core content about lambdas, is structured around that. By contrast, the AI version is a collection of loosely connected facts with an example. The important thing here is that even though the average reader isn’t going to break down the text in this way; they will subconsciously notice if something feels off, and it will impact their learning and retention negatively.
There are other differences as well, such as the lack of an example with an actual dataset in the GPT version. Such differences are not as severe — as they can be corrected with some prompting adjustments — but are still worth noting as an example of how AI often struggles with nuance and subtlety in its responses.
This brings me to my main point. It has been stated time and again that human creativity is not replaceable by machines. Here, I argue that same point, but in the specific context of this article. Why do we need human creativity specifically for coding tutorials?
Because a human walks you through the process as you are — a living, thinking, person trying to learn a confusing new concept — whereas a machine will at best give you just what it is, facts and no emotion or passion.
And these things matter, despite not appearing so at the surface. “Emotion” and “passion” may seem like words far removed from code, but each of the examples from my lambda example above fall under their banner. We just don’t always pay attention. Machines may feign emotion and passion with weighted words and a slew of adjectives, but at the end of the day, their writing simply won’t be the same.
And sometimes, that’s fine. I’ll be the first to admit it. If you just need some basic Python code to do a task — then fine, use ChatGPT. But this is fundamentally the same as going on Stack Overflow and copy-pasting code — we have been doing it for years, but we did not pretend such resources rendered coding tutorials obsolete. Why? Because these tutorials fill in the gaps that resources like this don’t give us. Long, detailed walk-throughs, not just with the common mistakes that GPT gives, but often the exact mistakes you run into when coding. Meaningful problems, not just with the example data that Claude generates, but with a real, fundamental issue a human was working on. And passionate writing, not just with the dry and fact-based directions Gemini provides, but with the same emotional investment in the task at hand that you yourself have. A human-written tutorial will give you these things.
AI will not.
This is not a limitation, but rather an opportunity. An opportunity for writers and readers of publications like Towards Data Science, if only we take a moment to recognize what it is we valued in the first place.
And that, I think, is a worthy endeavor indeed.
References
[1] https://www.edureka.co/blog/how-chatgpt-works-training-model-of-chatgpt/
[2] https://en.wikipedia.org/wiki/Functional_programming
The Death of Human-Written Code Tutorials in the ChatGPT Era … Or Not? was originally published in Towards Data Science on Medium, where people are continuing the conversation by highlighting and responding to this story.
from Datascience in Towards Data Science on Medium https://ift.tt/vbMicZ5
via IFTTT